import '@johnlindquist/kit'
import express, { Express, Request, Response } from 'express'
interface Script {
name: string
filePath: string}
const scripts = await getScripts()
const choices = scripts
.filter((s: Script) => s.name !== 'index')
.map((s: Script) => ({
name: s.name,
value: s.filePath,
description: s.filePath,
}))
const selectedScript = await arg<string>('Select a script to expose via REST API', choices)
const scriptName = selectedScript.split('/').pop()?.split('.')[0]
const port = await env('PORT', 'Enter the port to use for the REST API', '3000')
const baseUrl = `http://localhost:${port}`
const endpoint = `${baseUrl}/${scriptName}`
await div(
md(`
# REST API endpoint createdEndpoint: [${endpoint}](${endpoint})
## Example usage
\`\`\`bash
curl ${endpoint}
\`\`\`
## How to End Long-Running Scripts
Press "${cmd}+p" from the Main Menu to open the Process Manager. Select the process you want to end.
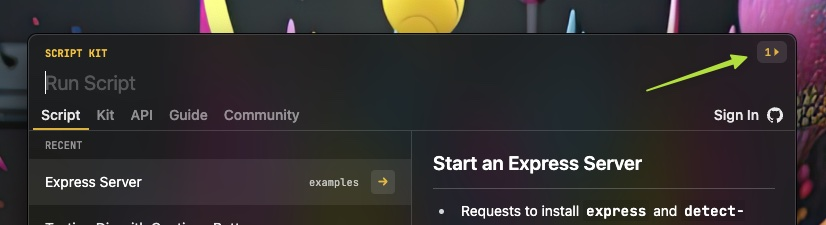
`)
)
hide()
const app: Express = express()
app.get(`/${scriptName}`, async (req: Request, res: Response) => {
try {
await run(selectedScript)
res.send(`Script ${scriptName} executed successfully`)
} catch (error: any) {
res.status(500).send(`Error executing script ${scriptName}: ${error}`)
}
})
await new Promise<void>((resolve, reject) => {
app.listen(port, () => resolve())
})